Write a program to reverse an array or string in C language.
Given an array (or string), the take is to reverse the array/string.
___________________________________________
STRING CODE
___________________________________________
Here is example of string:
Input = {AIR}
Output = {RIA}
Input = {RADHA}
Output = {AHDAR}
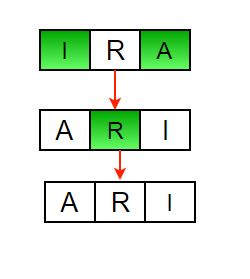
Here is code for reverse a string:
===========================================
#include <stdio.h>
void reverse(char* str) {
int i, j;
char temp;
for (i = 0, j = strlen(str) - 1; i < j; i++, j--) {
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
int main() {
char str[] = "Hello, world!";
reverse(str);
printf("%s\n", str);
return 0;
}
==================================================
This program takes a string as input, and reverses it by swapping the first and last characters, then the second and second to last characters, and so on, until the middle of the string is reached. The reversed string is then printed to the console.
___________________________________________
ARRAY CODE
___________________________________________
Here is example of Array:
Input = {1,2,3,4,5,6}
Output = {6,5,4,3,2,1}
Input = {2,4,6,8}
Output = {8,6,4,2}
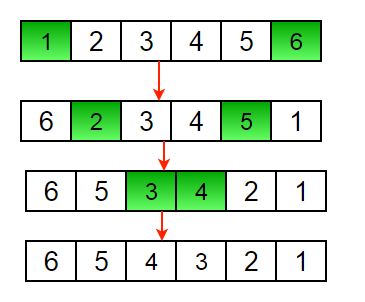
Here is code for reverse an Array:
==================================================
#include<string.h>
void reverse(int *arr, int start, int end) {
int temp;
while (start < end) {
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr)/sizeof(arr[0]);
reverse(arr, 0, n-1);
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
==================================================
This program takes an array and the start and end indices as input, and reverses the elements of the array by swapping the first and last elements, then the second and second to last elements, and so on, until the middle of the array is reached. The reversed array is then printed to the console.
0 Comments